

Unpacking tuples means assigning individual elements of a tuple to multiple variables.
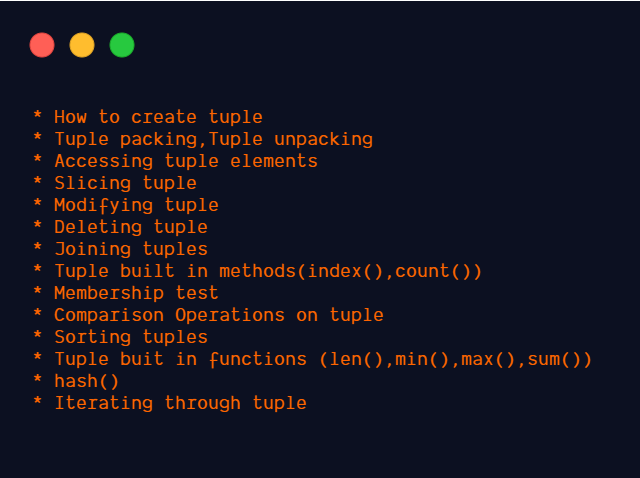
The Overflow Blog On the quantum internet, data doesn’t stream it teleports (Ep. Python uses the commas (,) to define a tuple, not parentheses. A tuple can be used for this purpose, whereas a list can’t be. Browse other questions tagged python-3.x list iterable-unpacking or ask your own question. There is another Python data type that you will encounter shortly called a dictionary, which requires as one of its components a value that is of an immutable type. If the values in the collection are meant to remain constant for the life of the program, using a tuple instead of a list guards against accidental modification. Sometimes you don’t want data to be modified. This operation is known as tuple packing: record 2, 'John', 'Doe', 'Sales' print(record) Wise versa, you can extract tuple values back into variables. A tuple can be created without using parentheses. (This is probably not going to be noticeable when the list or tuple is small.) defining tuple of one element a 1, alternative way a tuple(1) Packing and unpacking Python tuples. In tuple unpacking, the values in a tuple on the right are unpacked into the. In this case we used add function of operator module which simply adds the given list arguments to an empty list. Once Python has created a tuple in memory, it cannot be changed. Program execution is faster when manipulating a tuple than it is for the equivalent list. Approach 1 : Using reduce () reduce () is a classic list operation used to apply a particular function passed in its argument to all of the list elements. > t = ( 'foo', 'bar', 'baz', 'qux', 'quux', 'corge' ) > t = 'Bark!' Traceback (most recent call last):įile "", line 1, in t = 'Bark!' TypeError: 'tuple' object does not support item assignment For better understanding consider the following code. All values will be assigned to every variable on the left-hand side and all remaining values will be assigned to args. This means that there can be many number of arguments in place of (args) in python. In packing, we put values into a new tuple while in unpacking we extract those values into a single variable. Python uses a special syntax to pass optional arguments (args) for tuple unpacking. In another way, it is called unpacking of a tuple in Python. A given object can appear in a list multiple times: Unpacking a Tuple in Python: In Python, there is a feature of the tuple that assigns the right-hand side values to the left-hand side. (A list with a single object is sometimes referred to as a singleton list.) I also hope that my analogy with matrix rotation will help someone else get it faster in the future.> a = > a > a = > a > a = > a I decided to keep writing this Q&A to learn by explaining what I had discovered by experimenting, after not immediately getting the meaning of the answer I found while writing. This kind of variable assignment is the fundamental concept of unpacking in Python. Python has a very powerful tuple assignment feature that allows a tuple of variable names on the left of an assignment statement to be assigned values from a. As you can see, we’re assigning the three items inside of the mybox list to three variables item1, item2, item2.
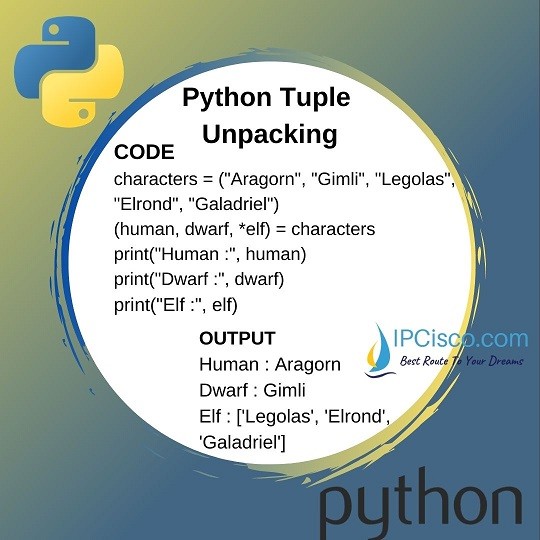
#PYTHON TUPLE UNPACKING CODE#
Let’s translate this same example into code for a better understanding: > mybox 'cables', 'headphones', 'USB' > item1, item2, item3 mybox. The last piece of the puzzle is to unpack the result and assign each tuple in its own variable using multiple assignment! l1 = Unpacking in Python is similar to unpack a box in real life. multiple variable assignment and tuple/list unpacking It is like rotating a matrix by providing the rows as arguments instead of the matrix itself. Tuples are immutable, and usually contain a heterogeneous sequence of elements that are accessed via unpacking (see later in this section) or indexing (or. Some pronounce it as though it were spelled too-ple (rhyming with Mott the Hoople), and others as though it were spelled tup-ple (rhyming with supple). Pronunciation varies depending on whom you ask. I now think of it as rotating values in a matrix 90° to make rows into columns and columns into rows. Python provides another type that is an ordered collection of objects, called a tuple.
#PYTHON TUPLE UNPACKING ZIP#
※ Note that if you use 3 lists of 4 elements, zip will output 4 lists of 3 elements l1 = We get 3 tuples of 2 elements, which are treated like 3 lists of 2 elements passed as arguments to zip: l1 = The key is unpacking the result of zip: print(*zip(l1,l2)) While I was trying to write my question clearly, I stumbled upon this answer Why does x,y = zip(*zip(a,b)) work in Python?, which gave me a hint that I still did not get before trying it in a REPL.
